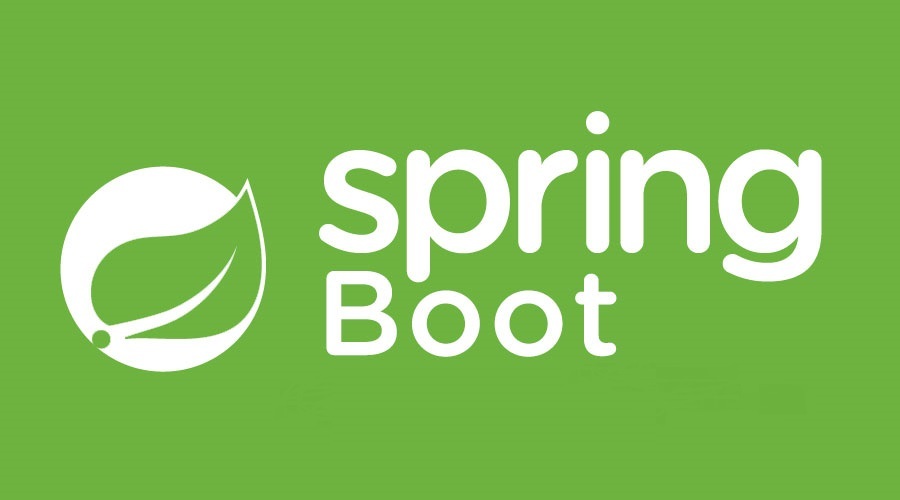
mapping
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
|
package hello.springmvc.basic.requetMapping;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import ch.qos.logback.classic.Logger;
@RestController // 뷰를 반환하는게 아니라 데이터 형태로 지원
public class MappingController {
private Logger log = (Logger) LoggerFactory.getLogger(getClass());
@RequestMapping("/hello-basic") // /hello-basic/ 이것도 가능
public String helloBasic() {
log.info("helloBasic");
return "ok";
}
@RequestMapping(value = "/mapping-get-v1",method = RequestMethod.GET)
public String mappingGetV1() {
log.info("mappingGetV1");
return "ok";
}
@GetMapping(value = "/mapping-get-v2")
public String mappinggetv2() {
log.info("mappinggetv2");
return "ok";
}
@GetMapping(value = "/mapping/{userid}")
public String mappingPath(@PathVariable("userid") String data) {
log.info("mappingpath userid={},data");
return "ok";
}
@GetMapping(value = "/mapping/users/{userid}/orders/{orderid}")
public String mappingPath(@PathVariable String userid,@PathVariable Long orderid) {
log.info("mappingPath userid={},orderid={},userid,orderid");
return "ok";
}
}
|
cs |
class mapping
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
package hello.springmvc.basic.requetMapping;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PatchMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/mapping/users")
public class mappingClassController {
@GetMapping
public String users() {
return "get users";
}
@PostMapping
public String adduser() {
return "post user";
}
@GetMapping("/{userid}")
public String findUser(@PathVariable String userid) {
return "get userid = " +userid;
}
@PatchMapping("/{userid}")
public String updateuser(@PathVariable String userid) {
return "update userid="+userid;
}
@DeleteMapping("/{userid}")
public String deleteuser(@PathVariable String userid) {
return "delete userid="+userid;
}
}
|
cs |
header 영역
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
package hello.springmvc.request;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpMethod;
import org.springframework.util.MultiValueMap;
import org.springframework.web.bind.annotation.CookieValue;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import ch.qos.logback.classic.Logger;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@RestController
public class RequestHeaderController {
private Logger log = (Logger) LoggerFactory.getLogger(getClass());
@RequestMapping("/headers")
public String header(HttpServletRequest request,
HttpServletResponse response,
HttpMethod httpMethod,
Locale locale,
@RequestHeader MultiValueMap<String, String> headerMap,
@RequestHeader("host") String host,
@CookieValue(value="MyCookie",required=false) String cookie) {
log.info("request={}",request);
log.info("response={}",response);
log.info("httpMethod={}",httpMethod);
log.info("locale={}",locale);
log.info("headerMap={}",headerMap);
log.info("myCookie={}",cookie);
return "ok";
}
}
|
cs |
request param
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
|
package hello.springmvc.request;
import java.io.IOException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import ch.qos.logback.classic.Logger;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class RequestParamController {
private Logger log = (Logger) LoggerFactory.getLogger(getClass());
@RequestMapping("/request-param-v1")
public void requestparamV1(HttpServletRequest request,HttpServletResponse response) throws IOException {
String username = request.getParameter("username");
int age = Integer.parseInt(request.getParameter("age"));
log.info("username={},age={}",username,age);
response.getWriter().write("ok");
}
@ResponseBody
@RequestMapping("/request-param-v2")
public String requestParamv2(@RequestParam("username") String memberName,
@RequestParam("age") int memberAge) {
log.info("username={} ,age={}",memberName,memberAge);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-v3")
public String requestparam(@RequestParam String username,@RequestParam int age) {
log.info("username={},age={}",username,age);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-v4")
public String requestparamV3(String username , int age) {
log.info("username={},age={}",username,age);
return "ok";
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
@ResponseBody
@RequestMapping("/request-param-required")
public String requestparamRequired(
@RequestParam(required = false) String username,
@RequestParam(required = false) int age
) {
log.info("username={},age={}",username,age);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-default")
public String requestparamRequired2(
@RequestParam(required = false,defaultValue = "guest") String username,
@RequestParam(required = false,defaultValue = "-1") int age
) {
log.info("username={},age={}",username,age);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-map")
public String requestparammap(
@RequestParam Map<String, Object> paramMap
) {
log.info("username={},age={}",paramMap.get("username"),paramMap.get("age"));
return "ok";
}
|
cs |
'기존 > 🏀Spring' 카테고리의 다른 글
(스프링소셜로그인)카카오 로그인 API (1) | 2022.07.06 |
---|---|
Java URLConnection 및 HttpURLConnection 사용 방법 (0) | 2022.07.06 |
[Spring] 로그인,관리자만들기 (0) | 2022.06.03 |
[Spring] 파일 업로드 (0) | 2022.06.03 |
[스프링-1] Spring 의 기본 속성 (2) | 2022.05.30 |
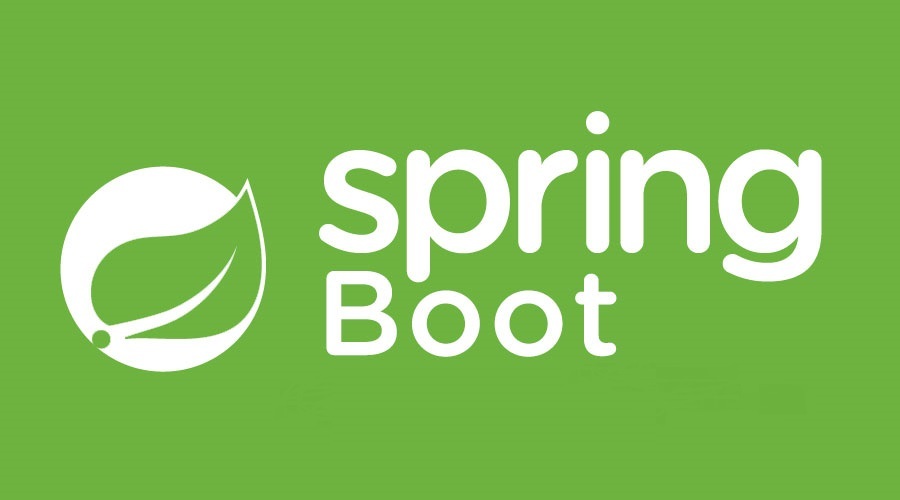
mapping
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
|
package hello.springmvc.basic.requetMapping;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import ch.qos.logback.classic.Logger;
@RestController // 뷰를 반환하는게 아니라 데이터 형태로 지원
public class MappingController {
private Logger log = (Logger) LoggerFactory.getLogger(getClass());
@RequestMapping("/hello-basic") // /hello-basic/ 이것도 가능
public String helloBasic() {
log.info("helloBasic");
return "ok";
}
@RequestMapping(value = "/mapping-get-v1",method = RequestMethod.GET)
public String mappingGetV1() {
log.info("mappingGetV1");
return "ok";
}
@GetMapping(value = "/mapping-get-v2")
public String mappinggetv2() {
log.info("mappinggetv2");
return "ok";
}
@GetMapping(value = "/mapping/{userid}")
public String mappingPath(@PathVariable("userid") String data) {
log.info("mappingpath userid={},data");
return "ok";
}
@GetMapping(value = "/mapping/users/{userid}/orders/{orderid}")
public String mappingPath(@PathVariable String userid,@PathVariable Long orderid) {
log.info("mappingPath userid={},orderid={},userid,orderid");
return "ok";
}
}
|
cs |
class mapping
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
package hello.springmvc.basic.requetMapping;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PatchMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/mapping/users")
public class mappingClassController {
@GetMapping
public String users() {
return "get users";
}
@PostMapping
public String adduser() {
return "post user";
}
@GetMapping("/{userid}")
public String findUser(@PathVariable String userid) {
return "get userid = " +userid;
}
@PatchMapping("/{userid}")
public String updateuser(@PathVariable String userid) {
return "update userid="+userid;
}
@DeleteMapping("/{userid}")
public String deleteuser(@PathVariable String userid) {
return "delete userid="+userid;
}
}
|
cs |
header 영역
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
package hello.springmvc.request;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpMethod;
import org.springframework.util.MultiValueMap;
import org.springframework.web.bind.annotation.CookieValue;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import ch.qos.logback.classic.Logger;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@RestController
public class RequestHeaderController {
private Logger log = (Logger) LoggerFactory.getLogger(getClass());
@RequestMapping("/headers")
public String header(HttpServletRequest request,
HttpServletResponse response,
HttpMethod httpMethod,
Locale locale,
@RequestHeader MultiValueMap<String, String> headerMap,
@RequestHeader("host") String host,
@CookieValue(value="MyCookie",required=false) String cookie) {
log.info("request={}",request);
log.info("response={}",response);
log.info("httpMethod={}",httpMethod);
log.info("locale={}",locale);
log.info("headerMap={}",headerMap);
log.info("myCookie={}",cookie);
return "ok";
}
}
|
cs |
request param
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
|
package hello.springmvc.request;
import java.io.IOException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import ch.qos.logback.classic.Logger;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Controller
public class RequestParamController {
private Logger log = (Logger) LoggerFactory.getLogger(getClass());
@RequestMapping("/request-param-v1")
public void requestparamV1(HttpServletRequest request,HttpServletResponse response) throws IOException {
String username = request.getParameter("username");
int age = Integer.parseInt(request.getParameter("age"));
log.info("username={},age={}",username,age);
response.getWriter().write("ok");
}
@ResponseBody
@RequestMapping("/request-param-v2")
public String requestParamv2(@RequestParam("username") String memberName,
@RequestParam("age") int memberAge) {
log.info("username={} ,age={}",memberName,memberAge);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-v3")
public String requestparam(@RequestParam String username,@RequestParam int age) {
log.info("username={},age={}",username,age);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-v4")
public String requestparamV3(String username , int age) {
log.info("username={},age={}",username,age);
return "ok";
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
@ResponseBody
@RequestMapping("/request-param-required")
public String requestparamRequired(
@RequestParam(required = false) String username,
@RequestParam(required = false) int age
) {
log.info("username={},age={}",username,age);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-default")
public String requestparamRequired2(
@RequestParam(required = false,defaultValue = "guest") String username,
@RequestParam(required = false,defaultValue = "-1") int age
) {
log.info("username={},age={}",username,age);
return "ok";
}
@ResponseBody
@RequestMapping("/request-param-map")
public String requestparammap(
@RequestParam Map<String, Object> paramMap
) {
log.info("username={},age={}",paramMap.get("username"),paramMap.get("age"));
return "ok";
}
|
cs |
'기존 > 🏀Spring' 카테고리의 다른 글
(스프링소셜로그인)카카오 로그인 API (1) | 2022.07.06 |
---|---|
Java URLConnection 및 HttpURLConnection 사용 방법 (0) | 2022.07.06 |
[Spring] 로그인,관리자만들기 (0) | 2022.06.03 |
[Spring] 파일 업로드 (0) | 2022.06.03 |
[스프링-1] Spring 의 기본 속성 (2) | 2022.05.30 |